Tutorial 6: Adding Airbridges#
Basic CPW Example#
Let’s start by creating a simple CPW and adding some airbridges to it.
[2]:
%load_ext autoreload
%autoreload 2
[3]:
import qiskit_metal as metal
from qiskit_metal import designs, draw
from qiskit_metal import MetalGUI, Dict, Headings
from qiskit_metal.qlibrary.terminations.open_to_ground import OpenToGround
from qiskit_metal.qlibrary.tlines.meandered import RouteMeander
[4]:
design = designs.DesignPlanar()
design.overwrite_enabled = True
gui = MetalGUI(design)
01:31PM 17s CRITICAL [_qt_message_handler]: line: 0, func: None(), file: None WARNING: Populating font family aliases took 255 ms. Replace uses of missing font family "Courier" with one that exists to avoid this cost.
[5]:
open_start_options = Dict(pos_x='1000um',
pos_y='0um',
orientation = '-90')
open_start_meander = OpenToGround(design,'Open_meander_start',options=open_start_options)
open_end_options = Dict(pos_x='1000um',
pos_y='1500um',
orientation='90',
termination_gap='10um')
open_end_meander = OpenToGround(design,'Open_meander_end',options=open_end_options)
meander_options = Dict(pin_inputs=Dict(start_pin=Dict(
component='Open_meander_start',
pin='open'),
end_pin=Dict(
component='Open_meander_end',
pin='open')
),
total_length='9mm',
fillet='99.99um')
meander = RouteMeander(design,'meander',options=meander_options)
01:31PM 17s WARNING [check_lengths]: For path table, component=meander, key=trace has short segments that could cause issues with fillet. Values in (1-1) are index(es) in shapely geometry.
01:31PM 17s WARNING [check_lengths]: For path table, component=meander, key=cut has short segments that could cause issues with fillet. Values in (1-1) are index(es) in shapely geometry.
[6]:
gui.rebuild()
gui.zoom_on_components(['meander'])
gui.screenshot()
01:31PM 20s WARNING [check_lengths]: For path table, component=meander, key=trace has short segments that could cause issues with fillet. Values in (1-1) are index(es) in shapely geometry.
01:31PM 20s WARNING [check_lengths]: For path table, component=meander, key=cut has short segments that could cause issues with fillet. Values in (1-1) are index(es) in shapely geometry.
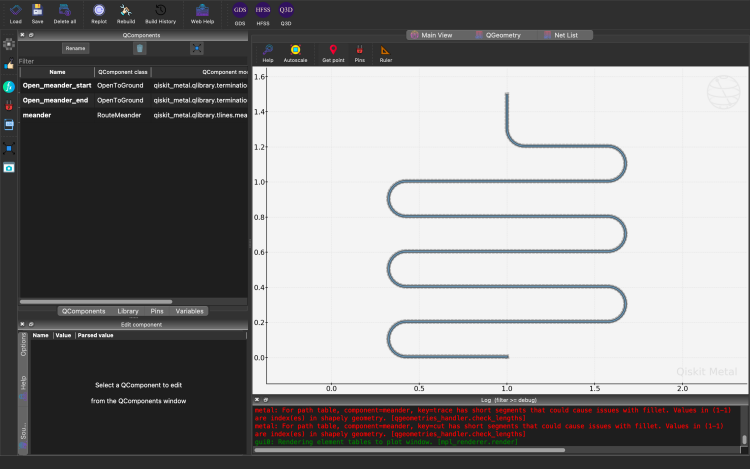
First we need to calculate the cross_over_length
and pitch
for the airbridge design.
[7]:
cpw_width = design.variables.cpw_width
cpw_gap = design.variables.cpw_gap
cpw_width, cpw_gap
[7]:
('10 um', '6 um')
[8]:
crossover_length = design.parse_value(cpw_width) + (2 * design.parse_value(cpw_gap))
crossover_length
[8]:
0.022
We’re going to make our crossover_length
slightly longer than the entirety of the cpw width + gap. That way when we’re on the turns of the meander, we can place our airbridges without having them intersect hte CPW.
[9]:
from squadds.components.airbridge.airbridge_generator import AirbridgeGenerator
[10]:
AirbridgeGenerator(
design=design,
target_comps=[meander],
crossover_length=[crossover_length],
min_spacing=0.005,
pitch=0.070,
add_curved_ab=True
)
gui.rebuild()
gui.zoom_on_components(['meander'])
gui.screenshot()
01:31PM 25s WARNING [check_lengths]: For path table, component=meander, key=trace has short segments that could cause issues with fillet. Values in (1-1) are index(es) in shapely geometry.
01:31PM 25s WARNING [check_lengths]: For path table, component=meander, key=cut has short segments that could cause issues with fillet. Values in (1-1) are index(es) in shapely geometry.
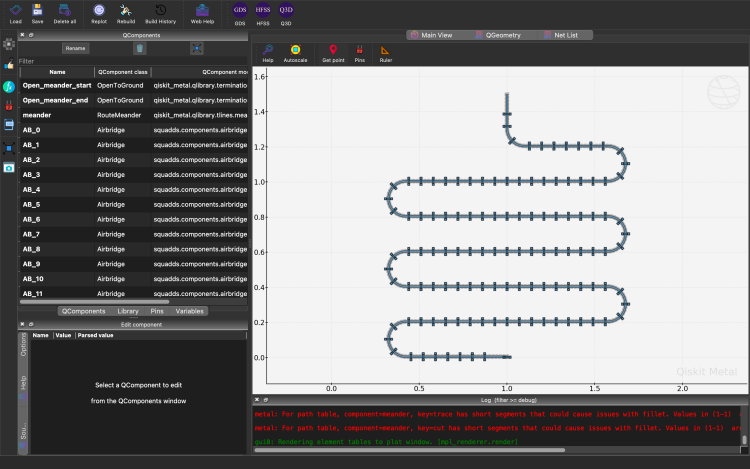
A high-level picture of what is happening on the backend.
You give
Airbridge_Generator()
atarget_cpw
. This is some cpw which you want to auto generate airbridges on.You give it it’s favorite options
cross_over_length
(thickness of bridge)min_spacing
(minimum spacing between bridges. This should be at least as large as your CPW’s gap)pitch
(spacing between the centers of each bridge)add_curved_ab
(if you want have airbridges on the turning points of your CPW) [default is True]
Note: Adding airbridges to the turning points of your CPW sometimes causes issue with the airbridges being too close to each other. In which case you may want to either set ``min_spacing`` higher or set ``add_curved_ab`` to False.
The generator then gets all the turning points of your CPW and calculates the directionality of them. It then uses a simplistic algorithm (I titled
find_ab_placement()
) to find where to place and their directionFinally the generator calls the original
squadds.components.airbridge.airbridge.py
and makes the airbridges in the locations specificed previously.
License#
This code is a part of SQuADDS
Developed by Sadman Ahmed Shanto
This tutorial is written by Sadman Ahmed Shanto
© Copyright Sadman Ahmed Shanto & Eli Levenson-Falk 2025.
This code is licensed under the MIT License. You may obtain a copy of this license in the LICENSE.txt file in the root directory of this source tree.
Any modifications or derivative works of this code must retain thiscopyright notice, and modified files need to carry a notice indicatingthat they have been altered from the originals.